XaGUI
Multiple pages
This example will create a GUI with 2 pages, both having 3 rows
Pages are counted from 1 in constructor and from 0 in methods
GuiMenu gui = xaGui.createMenu("&a&lExample", 3, 2);
gui.getCurrentPage(); // 1 (first page)
gui.getCurrentPageIndex(); // 0 (first page but indexed from 0)
gui.setSlot(1, Material.COAL); // The page here is not set, so it will be set to the current page which is 1 (0 indexed)
gui.setSlot(0, 2, Material.COAL); // Same as above, but slot 2 instead of 1
gui.setSlot(1, 0, Material.COAL); // Now we set COAL to slot 0 on page 2 (1 indexed)
gui.setSlot(1, 1, Material.CHARCOAL); // Now we set COAL to slot 0 on page 2 (1 indexed)
// Pages are indexed from 0 in all methods, such as .setSlot, .switchPage, etc.
gui.setSlot(0, 26, new GuiButton(new ItemStack(Material.ARROW)).setName("&aNext page").withListener((event) -> {
try {
gui.switchPage(gui.getCurrentPageIndex() + 1, (Player) event.getWhoClicked());
} catch (PageOutOfBoundException e) {
// IGNORE
}
}));
gui.setSlot(1, 18, new GuiButton(new ItemStack(Material.ARROW)).setName("&cGo back").withListener((event) -> {
try {
gui.switchPage(gui.getCurrentPageIndex() - 1, (Player) event.getWhoClicked());
} catch (PageOutOfBoundException e) {
// IGNORE
}
}));
gui.setOnPageSwitch((data) -> {
data.getPlayer().sendMessage("You switched to page " + data.getPage() + " from page " + data.getOldPage());
});
gui.open(player);
This is the result:
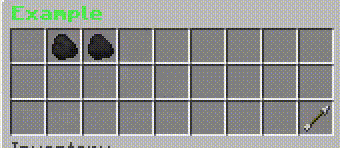